Longan Nano : I2C Master
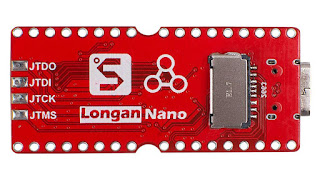
After SPI, serial and Gpio, the next building block is I2C/Master. That one is a bit peculiar. Overview/DMA It is actually a finite state machine. Each transition of a I2C transmission is associated with an event, with a corresponding "event" interrupt. The sequence is StartSent-AddressSent-DataDataData-BTC-Stop Simple enough. Where it becomes tricky is when you want to use DMA with it. You have to manage two interrupts source in parallel. It ends up as : 1- Do the beginning as usual, using plain I2C interrupt events. 2- When reaching the "data" stage, start a DMA transfer + disable I2C event interrupt (we trigger the DMA transfer only if the # of data is > 2, else we do it using basic interrupts) 3- When the DMA stage is done, re-enable the I2C event interrupts to finish the transfer To give an example: to refresh the SSD1306 screen in one step (1024 bytes), we only need ~ 6 interrupts ( 5 I2C + 1 DMA) rather than ~ 1030 interrupts in plain i2c interrupt